+ particle
parent
578e34e514
commit
b2f8fb6da8
33
README.md
33
README.md
|
@ -1,5 +1,38 @@
|
|||
|
||||
<p align="center">
|
||||
<img src="./examples/favicon.ico" alt="mark text" width="100" height="100">
|
||||
</p>
|
||||
|
||||
<h3 align="center">v-charts</h3>
|
||||
|
||||
<p align="center">
|
||||
<a href="https://travis-ci.org/ElemeFE/v-charts">
|
||||
<img src="https://travis-ci.org/ElemeFE/v-charts.svg?branch=master" alt="Build Status">
|
||||
</a>
|
||||
<a href="https://npmjs.org/package/v-charts">
|
||||
<img src="http://img.shields.io/npm/dm/v-charts.svg" alt="NPM downloads">
|
||||
</a>
|
||||
<a href="https://www.npmjs.org/package/v-charts">
|
||||
<img src="https://img.shields.io/npm/v/v-charts.svg" alt="Npm package">
|
||||
</a>
|
||||
<a>
|
||||
<img src="https://img.shields.io/badge/language-javascript-yellow.svg" alt="Language">
|
||||
</a>
|
||||
<a>
|
||||
<img src="https://img.shields.io/badge/license-MIT-000000.svg" alt="License">
|
||||
</a>
|
||||
<a href="https://gitter.im/ElemeFE/v-charts?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge">
|
||||
<img src="https://badges.gitter.im/ElemeFE/v-charts.svg" alt="Join the chat">
|
||||
</a>
|
||||
</p>
|
||||
|
||||
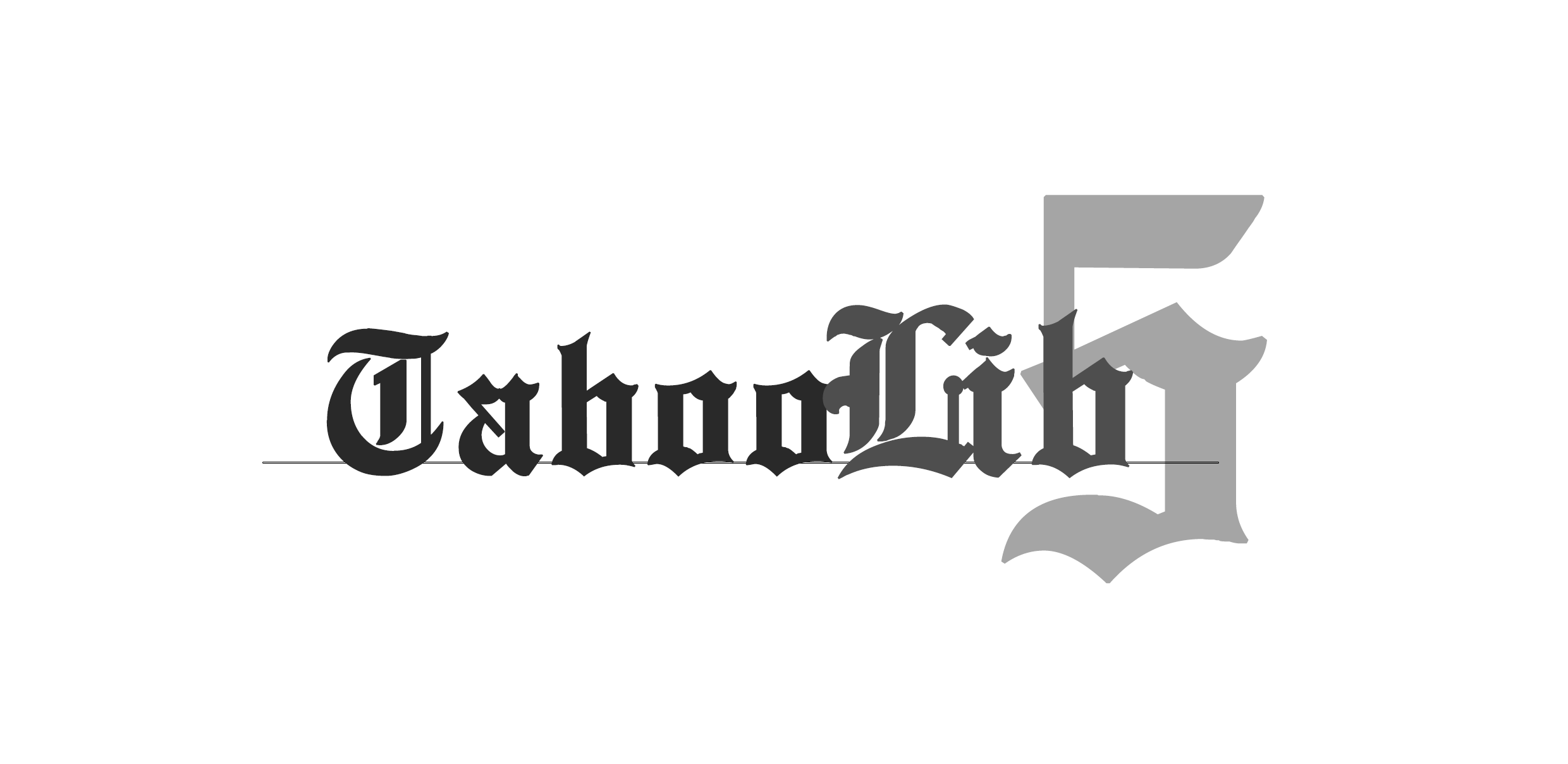
|
||||
|
||||

|
||||

|
||||

|
||||

|
||||
|
||||
插件文档
|
||||
---
|
||||
| 文档 | 地址 |
|
||||
|
|
|
@ -6,7 +6,7 @@ plugins {
|
|||
}
|
||||
|
||||
group = 'me.skymc'
|
||||
version = '5.07'
|
||||
version = '5.08'
|
||||
|
||||
sourceCompatibility = 1.8
|
||||
targetCompatibility = 1.8
|
||||
|
|
|
@ -0,0 +1,112 @@
|
|||
package io.izzel.taboolib.util;
|
||||
|
||||
import com.google.common.collect.Maps;
|
||||
import io.izzel.taboolib.util.lite.Numbers;
|
||||
import org.bukkit.util.NumberConversions;
|
||||
|
||||
import java.util.Arrays;
|
||||
import java.util.Map;
|
||||
import java.util.Objects;
|
||||
import java.util.regex.Matcher;
|
||||
import java.util.regex.Pattern;
|
||||
|
||||
/**
|
||||
* @Author sky
|
||||
* @Since 2019-10-06 17:18
|
||||
*/
|
||||
public class TMap {
|
||||
|
||||
private String name;
|
||||
private Map<String, String> content = Maps.newHashMap();
|
||||
|
||||
public TMap(String name) {
|
||||
this.name = name;
|
||||
}
|
||||
|
||||
public int getInt(String... key) {
|
||||
return getInt(key, 0);
|
||||
}
|
||||
|
||||
public int getInt(String[] key, int def) {
|
||||
return Arrays.stream(key).filter(content::containsKey).mapToInt(i -> NumberConversions.toInt(content.get(i))).findFirst().orElse(def);
|
||||
}
|
||||
|
||||
public double getDouble(String... key) {
|
||||
return getDouble(key, 0);
|
||||
}
|
||||
|
||||
public double getDouble(String[] key, double def) {
|
||||
return Arrays.stream(key).filter(content::containsKey).mapToDouble(i -> NumberConversions.toDouble(content.get(i))).findFirst().orElse(def);
|
||||
}
|
||||
|
||||
public boolean getBoolean(String... key) {
|
||||
return getBoolean(key, false);
|
||||
}
|
||||
|
||||
public boolean getBoolean(String[] key, boolean def) {
|
||||
return Arrays.stream(key).filter(content::containsKey).map(i -> Numbers.getBoolean(content.get(i))).findFirst().orElse(def);
|
||||
}
|
||||
|
||||
public String get(String... key) {
|
||||
return get(key, null);
|
||||
}
|
||||
|
||||
public String get(String[] key, String def) {
|
||||
return Arrays.stream(key).filter(content::containsKey).map(i -> content.get(i)).findFirst().orElse(def);
|
||||
}
|
||||
|
||||
public static TMap parse(String in) {
|
||||
Matcher matcher = Pattern.compile("(?<name>[^{}]+)?\\{(?<content>[^<>]+)}").matcher(in);
|
||||
if (matcher.find()) {
|
||||
TMap map = new TMap(matcher.group("name"));
|
||||
for (String content : matcher.group("content").split(";")) {
|
||||
String[] v = content.split("=");
|
||||
if (v.length == 2) {
|
||||
map.content.put(v[0].toLowerCase().trim(), v[1].trim());
|
||||
}
|
||||
}
|
||||
return map;
|
||||
}
|
||||
return new TMap(null);
|
||||
}
|
||||
|
||||
@Override
|
||||
public boolean equals(Object o) {
|
||||
if (this == o) {
|
||||
return true;
|
||||
}
|
||||
if (!(o instanceof TMap)) {
|
||||
return false;
|
||||
}
|
||||
TMap tMap = (TMap) o;
|
||||
return Objects.equals(getName(), tMap.getName()) &&
|
||||
Objects.equals(getContent(), tMap.getContent());
|
||||
}
|
||||
|
||||
@Override
|
||||
public int hashCode() {
|
||||
return Objects.hash(getName(), getContent());
|
||||
}
|
||||
|
||||
@Override
|
||||
public String toString() {
|
||||
return "TMap{" +
|
||||
"name='" + name + '\'' +
|
||||
", content=" + content +
|
||||
'}';
|
||||
}
|
||||
|
||||
// *********************************
|
||||
//
|
||||
// Getter and Setter
|
||||
//
|
||||
// *********************************
|
||||
|
||||
public String getName() {
|
||||
return name;
|
||||
}
|
||||
|
||||
public Map<String, String> getContent() {
|
||||
return content;
|
||||
}
|
||||
}
|
|
@ -0,0 +1,151 @@
|
|||
package io.izzel.taboolib.util.lite;
|
||||
|
||||
import com.google.common.collect.Lists;
|
||||
import io.izzel.taboolib.util.ArrayUtil;
|
||||
import io.izzel.taboolib.util.TMap;
|
||||
import io.izzel.taboolib.util.item.Items;
|
||||
import org.bukkit.Location;
|
||||
import org.bukkit.Particle;
|
||||
import org.bukkit.entity.Player;
|
||||
import org.bukkit.inventory.ItemStack;
|
||||
import org.bukkit.material.MaterialData;
|
||||
import org.bukkit.util.NumberConversions;
|
||||
|
||||
import java.util.Arrays;
|
||||
import java.util.List;
|
||||
import java.util.Map;
|
||||
import java.util.Optional;
|
||||
|
||||
/**
|
||||
* @Author sky
|
||||
* @Since 2019-10-06 1:02
|
||||
*/
|
||||
public class Effects {
|
||||
|
||||
private Particle particle;
|
||||
private Location center;
|
||||
private double[] offset = {0, 0, 0};
|
||||
private double speed = 0;
|
||||
private double range = 0;
|
||||
private int count = 0;
|
||||
private List<Player> player = Lists.newArrayList();
|
||||
private Object data;
|
||||
|
||||
public static Effects create(Particle particle, Location center) {
|
||||
return new Effects(particle, center);
|
||||
}
|
||||
|
||||
public static Effects parse(String in) {
|
||||
TMap map = TMap.parse(in);
|
||||
Effects effects = Effects.create(parseParticle(map.getName()), null);
|
||||
for (Map.Entry<String, String> entry : map.getContent().entrySet()) {
|
||||
switch (entry.getKey()) {
|
||||
case "offset":
|
||||
case "o":
|
||||
Double[] offset = Arrays.stream(entry.getValue().split(",")).map(NumberConversions::toDouble).toArray(Double[]::new);
|
||||
effects.offset(new double[] {offset.length > 0 ? offset[0] : 0, offset.length > 1 ? offset[1] : 0, offset.length > 2 ? offset[2] : 0});
|
||||
break;
|
||||
case "speed":
|
||||
case "s":
|
||||
effects.speed(NumberConversions.toDouble(entry.getValue()));
|
||||
break;
|
||||
case "range":
|
||||
case "r":
|
||||
effects.range(NumberConversions.toDouble(entry.getValue()));
|
||||
break;
|
||||
case "count":
|
||||
case "c":
|
||||
case "amount":
|
||||
case "a":
|
||||
effects.count(NumberConversions.toInt(entry.getValue()));
|
||||
break;
|
||||
case "data":
|
||||
case "d":
|
||||
String[] data = entry.getValue().split(":");
|
||||
if (effects.particle.getDataType().equals(ItemStack.class)) {
|
||||
effects.data(new ItemStack(Items.asMaterial(data[0]), 1, data.length > 1 ? NumberConversions.toShort(data[1]) : 0));
|
||||
} else if (effects.particle.getDataType().equals(MaterialData.class)) {
|
||||
effects.data(new MaterialData(Items.asMaterial(data[0]), data.length > 1 ? NumberConversions.toByte(data[1]) : 0));
|
||||
}
|
||||
break;
|
||||
}
|
||||
}
|
||||
return effects;
|
||||
}
|
||||
|
||||
public static Particle parseParticle(String in) {
|
||||
try {
|
||||
return Particle.valueOf(in.toUpperCase());
|
||||
} catch (Throwable ignored) {
|
||||
}
|
||||
return Particle.FLAME;
|
||||
}
|
||||
|
||||
Effects() {
|
||||
}
|
||||
|
||||
Effects(Particle particle, Location center) {
|
||||
this.particle = particle;
|
||||
this.center = center;
|
||||
}
|
||||
|
||||
public void play() {
|
||||
if (player.size() > 0) {
|
||||
player.forEach(p -> p.spawnParticle(particle, Optional.ofNullable(center).orElse(p.getLocation()), count, offset[0], offset[1], offset[2], speed, data));
|
||||
}
|
||||
if (range > 0 && center != null) {
|
||||
center.getWorld().getPlayers().stream().filter(p -> p.getLocation().distance(center) < range).forEach(p -> p.spawnParticle(particle, center, count, offset[0], offset[1], offset[2], speed, data));
|
||||
}
|
||||
}
|
||||
|
||||
public Effects particle(Particle particle) {
|
||||
this.particle = particle;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects center(Location center) {
|
||||
this.center = center;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects offset(double[] offset) {
|
||||
this.offset = offset;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects speed(double speed) {
|
||||
this.speed = speed;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects range(double range) {
|
||||
this.range = range;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects count(int count) {
|
||||
this.count = count;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects player(List<Player> player) {
|
||||
this.player = player;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects player(Player... player) {
|
||||
this.player = ArrayUtil.asList(player);
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects data(ItemStack data) {
|
||||
this.data = data;
|
||||
return this;
|
||||
}
|
||||
|
||||
public Effects data(MaterialData data) {
|
||||
this.data = data;
|
||||
return this;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,6 +1,7 @@
|
|||
package io.izzel.taboolib.util.lite;
|
||||
|
||||
import com.google.common.collect.Lists;
|
||||
import io.izzel.taboolib.util.Reflection;
|
||||
import org.bukkit.enchantments.Enchantment;
|
||||
import org.bukkit.entity.LivingEntity;
|
||||
import org.bukkit.entity.Player;
|
||||
|
@ -10,7 +11,6 @@ import org.bukkit.event.inventory.ClickType;
|
|||
import org.bukkit.event.inventory.InventoryClickEvent;
|
||||
import org.bukkit.inventory.ItemStack;
|
||||
|
||||
import java.lang.reflect.Field;
|
||||
import java.util.List;
|
||||
import java.util.Optional;
|
||||
|
||||
|
@ -22,11 +22,9 @@ public class Servers {
|
|||
|
||||
public static void setEnchantmentAcceptingNew(boolean value) {
|
||||
try {
|
||||
Field f = Enchantment.class.getDeclaredField("acceptingNew");
|
||||
f.setAccessible(true);
|
||||
f.set(null, value);
|
||||
} catch (Exception e) {
|
||||
e.printStackTrace();
|
||||
Reflection.setValue(null, Enchantment.class, true, "acceptingNew", value);
|
||||
} catch (Throwable t) {
|
||||
t.printStackTrace();
|
||||
}
|
||||
}
|
||||
|
||||
|
|
Loading…
Reference in New Issue